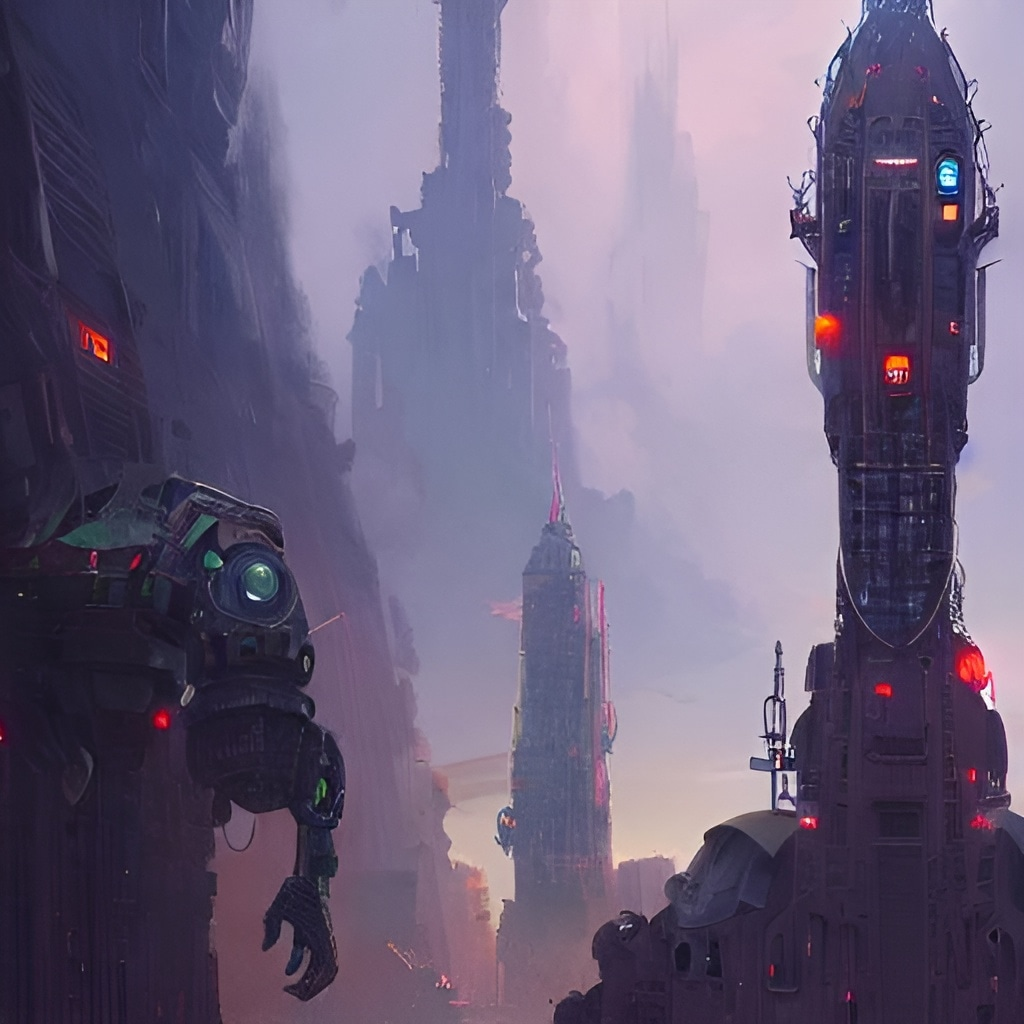
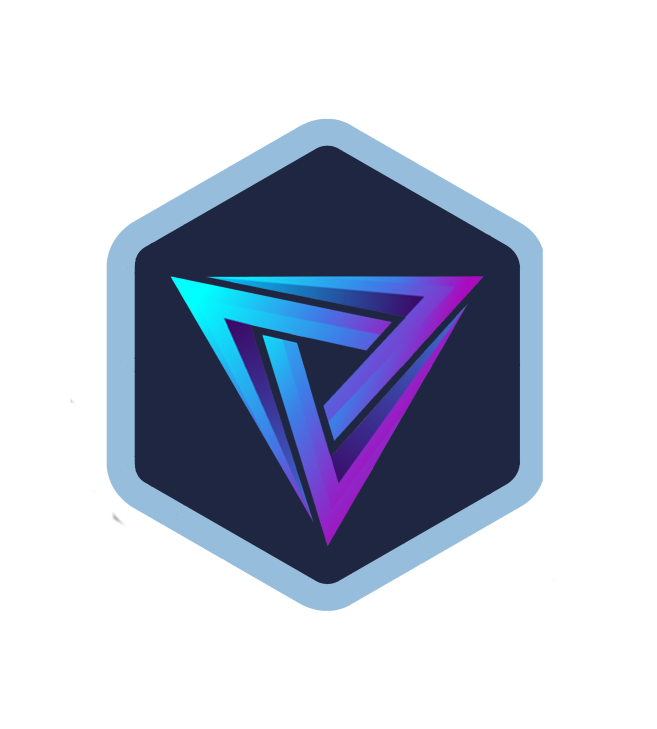
1 // SPDX-License-Identifier: MIT
2 pragma solidity ^0.8.9;
3
4 import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
5 import "@openzeppelin/contracts/token/ERC20/extensions/ERC20Burnable.sol";
6 import "@openzeppelin/contracts/token/ERC20/extensions/ERC20Snapshot.sol";
7 import "@openzeppelin/contracts/access/Ownable.sol";
8 import "@openzeppelin/contracts/security/Pausable.sol";
9 import "@openzeppelin/contracts/token/ERC20/extensions/ERC20Permit.sol";
10 import "@openzeppelin/contracts/token/ERC20/extensions/ERC20Votes.sol";
11 import "@openzeppelin/contracts/token/ERC20/extensions/ERC20FlashMint.sol";
12 contract VORDIUM is ERC20, ERC20Burnable, ERC20Snapshot, Ownable, Pausable, ERC20Permit, ERC20Votes, ERC20FlashMint {
13 constructor() ERC20("VORDIUM", "VORD") ERC20Permit("VORDIUM") {
14 _mint(msg.sender, 100000000 * 10 ** decimals());
15 }
16
17 function snapshot() public onlyOwner {
18 _snapshot();
19 }
20
21 function pause() public onlyOwner {
22 _pause();
23 }
24
25 function unpause() public onlyOwner {
26 _unpause();
27 }
28
29 function mint(address to, uint256 amount) public onlyOwner {
30 _mint(to, amount);
31 }
32
33 function _beforeTokenTransfer(address from, address to, uint256 amount)
34 internal
35 whenNotPaused
36 override(ERC20, ERC20Snapshot){
37 super._beforeTokenTransfer(from, to, amount);
38 }
39
40 // The following functions are overrides required by Solidity.
41
42 function _afterTokenTransfer(address from, address to, uint256 amount)
43 internal
44 override(ERC20, ERC20Votes){
45 super._afterTokenTransfer(from, to, amount);
46 }
47
48 function _mint(address to, uint256 amount)
49 internal
50 override(ERC20, ERC20Votes){
51 super._mint(to, amount);
52 }
53
54 function _burn(address account, uint256 amount)
55 internal
56 override(ERC20, ERC20Votes){
57 super._burn(account, amount);
58 }
59 }
SolidityAbout
Ai.vordium.com is an AI-powered Solidity Smart Contract Auditor that uses machine learning to analyze code for vulnerabilities, errors, and issues. The state-of-the-art technology generates comprehensive reports that details any issues found, including a description of the problem, the location of the problem in the code, and suggestions for how to fix it.
Investors in VORD could benefit from the increased security of the Ethereum network and demand for the native token ($VORD). As more and more organizations adopt blockchain technology, the need for secure and reliable smart contract solutions will increase. By investing in Vordium.com, investors can capitalize on this trend and potentially see significant returns.
In addition to providing a valuable service to investors, vordium.com could lead to more mainstream adoption of blockchain technology. As smart contracts become more secure, organizations and individuals will be more likely to use them for various applications. This could include financial transactions to supply chain management, creating new opportunities for businesses and individuals. Overall, Ai.vordium.com is a promising technology that has the potential to drive mainstream adoption of blockchain technology and benefit investors in the process.
Theory of Action
Machine learning
The auditor uses machine learning algorithms to identify patterns and trends in the code that could indicate potential issues.Report generation
The auditor generates a report detailing any issues found, and suggestions for how to fix them before deployment on the blockchain.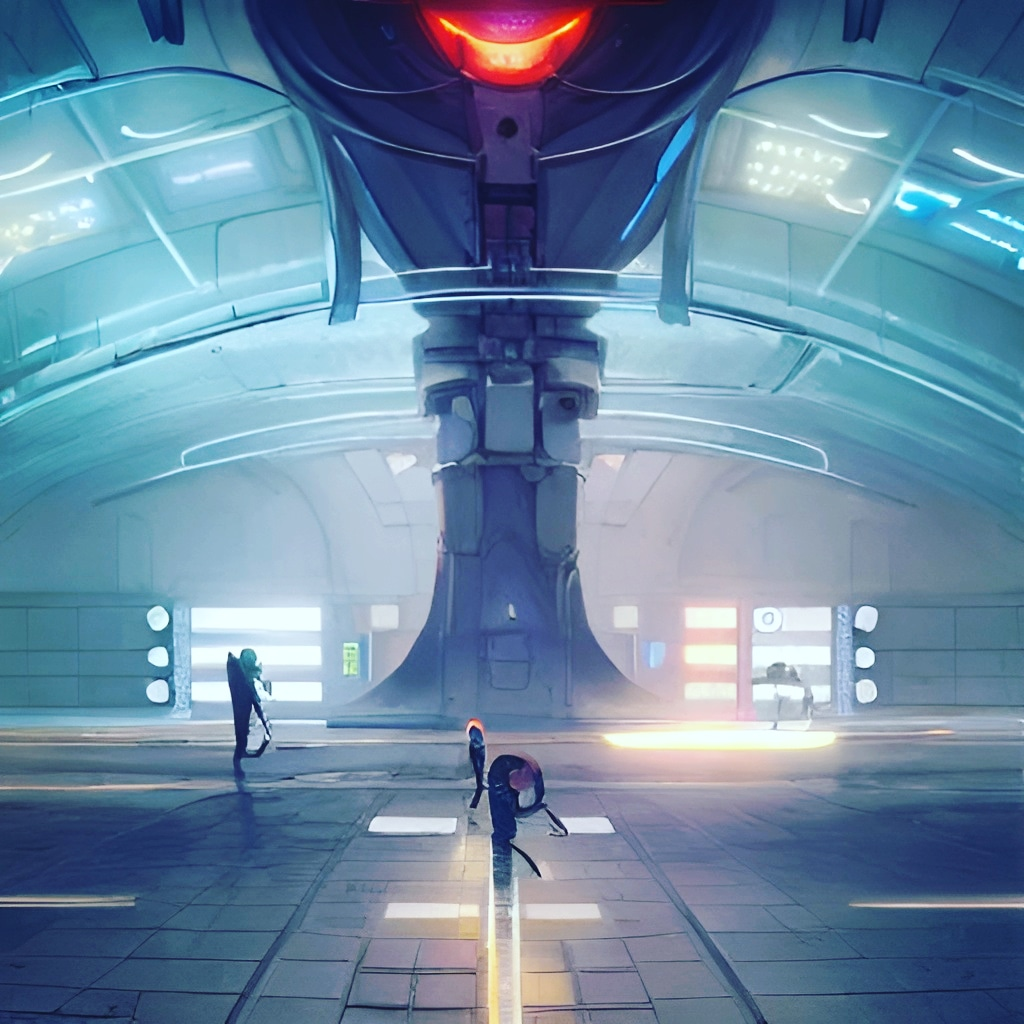